I found the below code for batch printing.
The only problem seems to be that it will only plot everything on 11x17 as it is set to Tabloid. Which is good for all of our drawings except for the BOM sheets. We print our BOMs on 8.5 x 11.
Worst case scenario is we toss a few sheets out each time we batch print. If possible I would rather change it to one of two options. If one of the following options is not too difficult of a change, could someone please fix this?
1) It would automatically change and print the BOMs on the letter size paper.
If that cant be done too easily, then possibly this...
2) It skips the BOMs in the printing process entirely. It is possible to have a dozen or more Bom sheets in a file, though its rare.
The only problem seems to be that it will only plot everything on 11x17 as it is set to Tabloid. Which is good for all of our drawings except for the BOM sheets. We print our BOMs on 8.5 x 11.
Worst case scenario is we toss a few sheets out each time we batch print. If possible I would rather change it to one of two options. If one of the following options is not too difficult of a change, could someone please fix this?
1) It would automatically change and print the BOMs on the letter size paper.
If that cant be done too easily, then possibly this...
2) It skips the BOMs in the printing process entirely. It is possible to have a dozen or more Bom sheets in a file, though its rare.
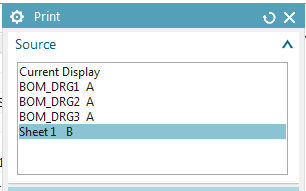
Code:
Option Strict Off
Imports System
Imports System.Windows.Forms
Imports NXOpen
Module Module1
Dim theSession As Session = Session.GetSession()
Dim defaultPrinterName As String = ""
Sub Main()
Dim workPart As Part = theSession.Parts.Work
Dim lw As ListingWindow = theSession.ListingWindow
'lw.Open()
Dim printerSettings As New System.Drawing.Printing.PrinterSettings
Try
defaultPrinterName = printerSettings.PrinterName
Catch ex As System.Exception
defaultPrinterName = ""
Finally
printerSettings = Nothing
End Try
If String.IsNullOrEmpty(defaultPrinterName) Then
MessageBox.Show("No default printer found.", "Printer error", MessageBoxButtons.OK, MessageBoxIcon.Error)
Return
End If
For Each tempPart As Part In theSession.Parts
If tempPart.IsFullyLoaded Then
'lw.WriteLine("part: " & tempPart.FullPath)
ChangeDisplayPart(tempPart)
For Each tempSheet As Drawings.DrawingSheet In tempPart.DrawingSheets
'lw.WriteLine(" sheet: " & tempSheet.Name)
tempPart.DraftingViews.UpdateViews(Drawings.DraftingViewCollection.ViewUpdateOption.OutOfDate, tempSheet)
PrintSheet(tempPart, tempSheet)
Next
'lw.WriteLine("")
End If
ChangeDisplayPart(workPart)
Next
'lw.Close()
End Sub
Sub PrintSheet(ByVal thePart As Part, ByVal theSheet As Drawings.DrawingSheet)
Dim printBuilder1 As PrintBuilder
printBuilder1 = thePart.PlotManager.CreatePrintBuilder()
printBuilder1.PrinterText = defaultPrinterName
'printer settings, change as desired
printBuilder1.Copies = 1
printBuilder1.ThinWidth = 1.0
printBuilder1.NormalWidth = 2.0
printBuilder1.ThickWidth = 3.0
printBuilder1.Output = PrintBuilder.OutputOption.WireframeBlackWhite
printBuilder1.RasterImages = True
printBuilder1.Orientation = PrintBuilder.OrientationOption.Landscape
'printBuilder1.Paper = PrintBuilder.PaperSize.Letter
'printBuilder1.Paper = PrintBuilder.PaperSize.A4
'printBuilder1.Paper = PrintBuilder.PaperSize.11X17
printBuilder1.Paper = PrintBuilder.PaperSize.Tabloid
Dim paper1 As PrintBuilder.PaperSize
paper1 = printBuilder1.Paper
Dim sheets1(0) As NXObject
sheets1(0) = theSheet
printBuilder1.SourceBuilder.SetSheets(sheets1)
Dim nXObject1 As NXObject
Try
nXObject1 = printBuilder1.Commit()
Catch ex As NXException
'optional: add code to log/display error
Finally
' printBuilder1.Destroy()
End Try
End Sub
Public Sub ChangeDisplayPart(ByVal myPart As Part)
'make the given component the display part
Dim markId1 As Session.UndoMarkId
markId1 = theSession.SetUndoMark(Session.MarkVisibility.Invisible, "Display Part")
Try
Dim partLoadStatus1 As PartLoadStatus
Dim status1 As PartCollection.SdpsStatus
status1 = theSession.Parts.SetDisplay(myPart, False, False, partLoadStatus1)
partLoadStatus1.Dispose()
Catch ex As NXException
'optional: add code to log/display error
theSession.UndoToMark(markId1, "Display Part")
Finally
theSession.DeleteUndoMark(markId1, "Display Part")
End Try
End Sub
End Module