CAD_ROB
Aerospace
- Feb 3, 2022
- 13
Hi, Im after some advice whether its possible to create a macro in Catia V5 to take a product with a number of .catparts, those catparts have a partbody that are published. I want the macro to be able to generate a new catpart in a different product and within that new .catpart have the published partbody link from the other .catpart from the other product. See Image with this post. So from the list Item 0010 would become 0110 with the published Partbody from 0010.
Any advice is welcome... Thanks.
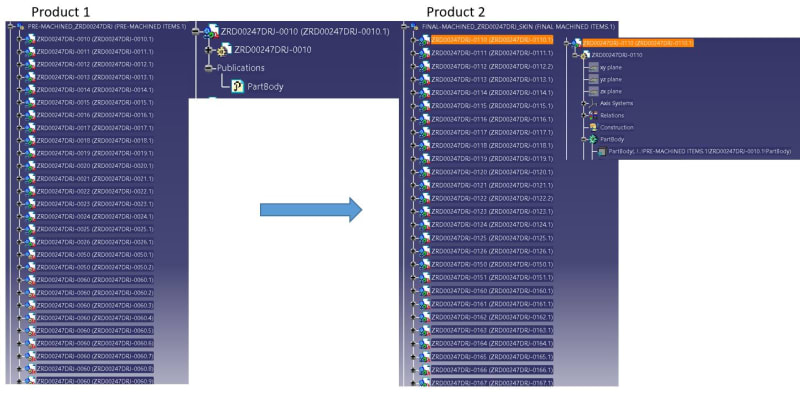
Any advice is welcome... Thanks.