Barnon
Mechanical
- Jul 23, 2016
- 97
I'm trying to write a Journal that would create a Knurl on any cylindrical face, like shown below, with just a few inputs. Manually I create a plane tangent to the face, sketch a curve, wrap it to face, create another sketch, sweep that sketch with the wrapped curve, mirror sweep and then a circular pattern.
I'm new to Journaling and the code below just creates the first plane tangent to the face. It works when my geometry is relative to absolute but I want it to work relative to WCS. Is there any command to make that happen? I've seen Csys.MapPoint in this forum but seems like I should be able to always work off the WCS.
Option Strict Off
Imports System
Imports NXOpen
Imports NXOpen.UF
Module Module1
Sub Main()
Dim theSession As Session = Session.GetSession()
Dim theUfSession As UFSession = UFSession.GetUFSession()
If IsNothing(theSession.Parts.Work) Then
'active part required
Return
End If
Dim workPart As Part = theSession.Parts.Work
Dim lw As ListingWindow = theSession.ListingWindow
lw.Open()
Const undoMarkName As String = "NXJ journal"
Dim markId1 As Session.UndoMarkId
markId1 = theSession.SetUndoMark(Session.MarkVisibility.Visible, undoMarkName)
Dim myFace As Face
If SelectEdge("select circular edge", myFace) = Selection.Response.Cancel Then
Return
End If
Dim ufs As NXOpen.UF.UFSession = NXOpen.UF.UFSession.GetUFSession
Dim axisPoint(2) As Double
Dim axisVector(2) As Double
Dim box(5) As Double 'minX, minY, minZ, maxX, maxY, maxZ.
Dim surfType As Integer
Dim r1, r2 As Double
Dim flip As Integer
ufs.Modl.AskFaceData(myFace.Tag, surfType, axisPoint, axisVector, box, r1, r2, flip)
Dim origin As New NXOpen.Point3d(box(3),0,box(5)/2)
Dim axisX As New NXOpen.Point3d(box(3),0,box(5)/2-1)
Dim wcsMatrix As NXOpen.Matrix3x3 = workPart.WCS.CoordinateSystem.Orientation.Element
Dim sketchPlane As NXOpen.DatumPlane = workPart.Datums.CreateFixedDatumPlane(origin, wcsMatrix)
Dim horizAxis As NXOpen.DatumAxis = workPart.Datums.CreateFixedDatumAxis(origin, axisX)
End Sub
Function SelectEdge(ByVal prompt As String, ByRef selObj As TaggedObject) As Selection.Response
Dim theUI As UI = UI.GetUI
Dim title As String = "Select circular edge"
Dim includeFeatures As Boolean = False
Dim keepHighlighted As Boolean = False
Dim selAction As Selection.SelectionAction = Selection.SelectionAction.ClearAndEnableSpecific
Dim cursor As Point3d
Dim scope As Selection.SelectionScope = Selection.SelectionScope.WorkPart
Dim selectionMask_array(0) As Selection.MaskTriple
With selectionMask_array(0)
.Type = UFConstants.UF_solid_type
.SolidBodySubtype = UFConstants.UF_UI_SEL_FEATURE_CYLINDRICAL_FACE
End With
Dim resp As Selection.Response = theUI.SelectionManager.SelectTaggedObject(prompt, _
title, scope, selAction, _
includeFeatures, keepHighlighted, selectionMask_array, _
selobj, cursor)
If resp = Selection.Response.ObjectSelected OrElse resp = Selection.Response.ObjectSelectedByName Then
Return Selection.Response.Ok
Else
Return Selection.Response.Cancel
End If
End Function
End Module
I'm new to Journaling and the code below just creates the first plane tangent to the face. It works when my geometry is relative to absolute but I want it to work relative to WCS. Is there any command to make that happen? I've seen Csys.MapPoint in this forum but seems like I should be able to always work off the WCS.
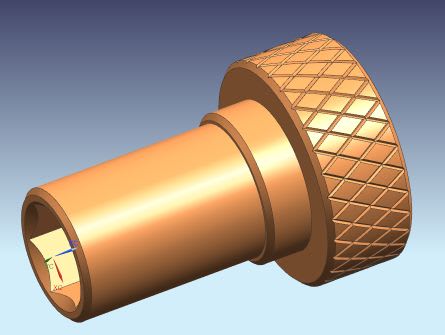
Option Strict Off
Imports System
Imports NXOpen
Imports NXOpen.UF
Module Module1
Sub Main()
Dim theSession As Session = Session.GetSession()
Dim theUfSession As UFSession = UFSession.GetUFSession()
If IsNothing(theSession.Parts.Work) Then
'active part required
Return
End If
Dim workPart As Part = theSession.Parts.Work
Dim lw As ListingWindow = theSession.ListingWindow
lw.Open()
Const undoMarkName As String = "NXJ journal"
Dim markId1 As Session.UndoMarkId
markId1 = theSession.SetUndoMark(Session.MarkVisibility.Visible, undoMarkName)
Dim myFace As Face
If SelectEdge("select circular edge", myFace) = Selection.Response.Cancel Then
Return
End If
Dim ufs As NXOpen.UF.UFSession = NXOpen.UF.UFSession.GetUFSession
Dim axisPoint(2) As Double
Dim axisVector(2) As Double
Dim box(5) As Double 'minX, minY, minZ, maxX, maxY, maxZ.
Dim surfType As Integer
Dim r1, r2 As Double
Dim flip As Integer
ufs.Modl.AskFaceData(myFace.Tag, surfType, axisPoint, axisVector, box, r1, r2, flip)
Dim origin As New NXOpen.Point3d(box(3),0,box(5)/2)
Dim axisX As New NXOpen.Point3d(box(3),0,box(5)/2-1)
Dim wcsMatrix As NXOpen.Matrix3x3 = workPart.WCS.CoordinateSystem.Orientation.Element
Dim sketchPlane As NXOpen.DatumPlane = workPart.Datums.CreateFixedDatumPlane(origin, wcsMatrix)
Dim horizAxis As NXOpen.DatumAxis = workPart.Datums.CreateFixedDatumAxis(origin, axisX)
End Sub
Function SelectEdge(ByVal prompt As String, ByRef selObj As TaggedObject) As Selection.Response
Dim theUI As UI = UI.GetUI
Dim title As String = "Select circular edge"
Dim includeFeatures As Boolean = False
Dim keepHighlighted As Boolean = False
Dim selAction As Selection.SelectionAction = Selection.SelectionAction.ClearAndEnableSpecific
Dim cursor As Point3d
Dim scope As Selection.SelectionScope = Selection.SelectionScope.WorkPart
Dim selectionMask_array(0) As Selection.MaskTriple
With selectionMask_array(0)
.Type = UFConstants.UF_solid_type
.SolidBodySubtype = UFConstants.UF_UI_SEL_FEATURE_CYLINDRICAL_FACE
End With
Dim resp As Selection.Response = theUI.SelectionManager.SelectTaggedObject(prompt, _
title, scope, selAction, _
includeFeatures, keepHighlighted, selectionMask_array, _
selobj, cursor)
If resp = Selection.Response.ObjectSelected OrElse resp = Selection.Response.ObjectSelectedByName Then
Return Selection.Response.Ok
Else
Return Selection.Response.Cancel
End If
End Function
End Module