pittbuck
Computer
- May 1, 2017
- 31
I am trying to write a journal that will export a single object from a specified layer to a .stl file. I've tried to "rewrite" the journal that is on the following website, but it doesn't seem to help. Maybe I am re-writing the code wrong (very likely).
This file needs to go to a specified place and needs a specified name, but I'll worry about that later. If this file goes into the TEMP folder on my computer for now, that's fine. When export the object to an STL file, the following has to be in the "rapid prototyping" window:
.
Thanks in advance for any advice.
This file needs to go to a specified place and needs a specified name, but I'll worry about that later. If this file goes into the TEMP folder on my computer for now, that's fine. When export the object to an STL file, the following has to be in the "rapid prototyping" window:
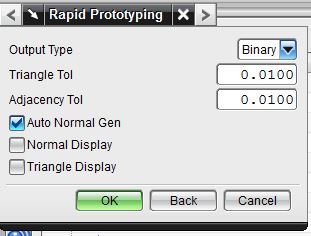
Thanks in advance for any advice.