Hello everyone!
I'm trying to automate a process in CATIA v5 using macros.
I have a product with other products or parts underneath it. My goal is to create one folder with root product's part number as name, and in this folder, I'm going to save all parts that are directly under the root product, and for each product, under the root product, I'm going to create another folder in a way similar to the root product.
To help me explain, an example of the product may be this:
In this, the root product is product1, that has product2, product3, and part1 underneath it. So my macro aims to create a folder where part1 will be saved and two more folders will be created (with names product2 and product3). Subsequently, part2 will be saved in product2 folder and part3 and part4 will be saved in product3 folder.
I've managed to create a macro that scrolls down the tree and create when needed the folder. But I have problems to save the files.
In fact, SaveAs property works, as I know, only with document object, and with this macro, I get only products and parts.
I'm new to coding macros but here's the code:
Sub CATMain()
Dim oProdDoc As ProductDocument
Set oProdDoc = CATIA.ActiveDocument
Dim oRootProd As Product
Set oRootProd = oProdDoc.Product
Dim RootPath As String
Dim initialPath As String
'this is the path where the root product's folder will be created
initialPath = InputBox("Copia ed Incolla il percorso dove andranno salvati: ")
'changing PN in PN_REV
oRootProd.PartNumber = oRootProd.PartNumber & "_" & oRootProd.Revision
'creation of the root product's folder
RootPath = initialPath & "\" & oRootProd.PartNumber
MkDir RootPath
'using a recursive sub, scroll down the tree
Call WalkDownTree(oRootProd, RootPath)
End Sub
'--------------------------------------------------------------
Sub WalkDownTree(oRootProd As Product, RootPath As String)
Dim oProds As Products
Set oProds = oRootProd.Products
Dim i As Integer
For i = 1 To oProds.Count
'we have a produc or a part?
Dim parentFileName As String
parentFileName = oProds.Item(i).ReferenceProduct.Parent.Name
'if I have a product
If InStr(parentFileName, ".CATProduct") <> 0 Then
'changing PN in PN_REV
oProds.Item(i).PartNumber = oProds.Item(i).PartNumber & "_" & oProds.Item(i).Revision
'creating the folder of the product under the root product
Dim finalPath As String
finalPath = RootPath & "\" & oProds.Item(i).PartNumber
MkDir finalPath
'because it is a product it has some items under it, so I have to recall the sub
Call WalkDownTree(oProds.Item(i), RootPath)
Else
'I have a part
'changing PN in PN_REV
oProds.Item(i).PartNumber = oProds.Item(i).PartNumber & "_" & oProds.Item(i).Revision
End If
Next
End Sub
So is there a possibility to get the document from parts and products?
Any help will be very appreciated!
Thanks
I'm trying to automate a process in CATIA v5 using macros.
I have a product with other products or parts underneath it. My goal is to create one folder with root product's part number as name, and in this folder, I'm going to save all parts that are directly under the root product, and for each product, under the root product, I'm going to create another folder in a way similar to the root product.
To help me explain, an example of the product may be this:
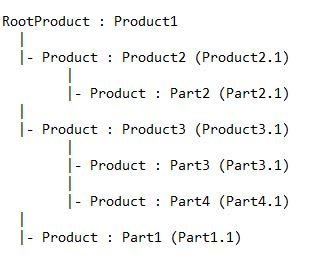
In this, the root product is product1, that has product2, product3, and part1 underneath it. So my macro aims to create a folder where part1 will be saved and two more folders will be created (with names product2 and product3). Subsequently, part2 will be saved in product2 folder and part3 and part4 will be saved in product3 folder.
I've managed to create a macro that scrolls down the tree and create when needed the folder. But I have problems to save the files.
In fact, SaveAs property works, as I know, only with document object, and with this macro, I get only products and parts.
I'm new to coding macros but here's the code:
Sub CATMain()
Dim oProdDoc As ProductDocument
Set oProdDoc = CATIA.ActiveDocument
Dim oRootProd As Product
Set oRootProd = oProdDoc.Product
Dim RootPath As String
Dim initialPath As String
'this is the path where the root product's folder will be created
initialPath = InputBox("Copia ed Incolla il percorso dove andranno salvati: ")
'changing PN in PN_REV
oRootProd.PartNumber = oRootProd.PartNumber & "_" & oRootProd.Revision
'creation of the root product's folder
RootPath = initialPath & "\" & oRootProd.PartNumber
MkDir RootPath
'using a recursive sub, scroll down the tree
Call WalkDownTree(oRootProd, RootPath)
End Sub
'--------------------------------------------------------------
Sub WalkDownTree(oRootProd As Product, RootPath As String)
Dim oProds As Products
Set oProds = oRootProd.Products
Dim i As Integer
For i = 1 To oProds.Count
'we have a produc or a part?
Dim parentFileName As String
parentFileName = oProds.Item(i).ReferenceProduct.Parent.Name
'if I have a product
If InStr(parentFileName, ".CATProduct") <> 0 Then
'changing PN in PN_REV
oProds.Item(i).PartNumber = oProds.Item(i).PartNumber & "_" & oProds.Item(i).Revision
'creating the folder of the product under the root product
Dim finalPath As String
finalPath = RootPath & "\" & oProds.Item(i).PartNumber
MkDir finalPath
'because it is a product it has some items under it, so I have to recall the sub
Call WalkDownTree(oProds.Item(i), RootPath)
Else
'I have a part
'changing PN in PN_REV
oProds.Item(i).PartNumber = oProds.Item(i).PartNumber & "_" & oProds.Item(i).Revision
End If
Next
End Sub
So is there a possibility to get the document from parts and products?
Any help will be very appreciated!
Thanks